Raspberry Pi powered temp/humidity/moisture monitoring system:
TLDR: setting up a Raspberry Pi to monitor temperature, humidity, soil moisture and run a camera.
So currently running Raspbian GNU/Linux 10 (buster) on a Pi Model 3B with 1GB memory although it could probably run off Pi Zero WH ($16.58 USD). Any would do, as long as it has GPIO to connect the sensors and in my case, the camera connector.
Hooked upto this is a camera, a temp/humidity sensor and a capacitive moisture/temp sensor (most available from PiHut). I think it's not polite to link in forum so I've included model no's etc for your googling pleasure
Humidity/Temp:
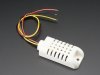
AM2302 (wired DHT22) temperature-humidity sensor ADA393 [AM2302] - $17.85 USD
Soil sensor:
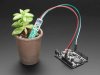
Adafruit STEMMA Soil Sensor - I2C Capacitive Moisture Sensor [ADA4026] - $9.56 USD
Camera:
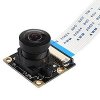
SainSmart Wide Angle Fish-Eye Camera Lenses for Raspberry Pi Arduino (approx $27 USD from amazon)
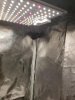
Wiring:
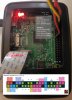
Hooking it all up:
I'll start with the two sensors.
Quick version:
Use python script to obtain readings every (x) seconds and send/save readings somewhere.
Run python script as a service so it runs on boot ..24/7
What you do with the readings can obviously vary a lot. Save them to a text file, a database etc on the Pi SD card or send them somewhere else over wifi/network.
Personally I prefer not relying on the SD card as they can be prone to failure and I wanted to be able to monitor them using apps I felt were a bit too demanding for running on the Pi (you could run them on the Pi though).
I instead opted to send the readings over http to InfluxDB which runs on a small linux NAS box at home. This means the Pi just has to get the readings and make an http request with the values which I can then view as my fancy looking dashboard.
There are quite a few good tutorials and variations on setting this up if you search for "am2302 raspberry pi influxdb"
The script I run for both sensors.
There are a few things to install which the script will need. To install them, run the following from the Pi's terminal. [Disclaimer: following is not complete at present]
NB: when installing, you will be asked to confirm Y/N.
So first, create a new python script (called mine sensor.py) and saved it in a new folder (tent) under the default Pi user's home dir (/home/Pi/tent)
Once you have saved your script. The next step is to run it as a service.
Then paste the following into the file.
You can save and exit the nano editor using [CTRL-X], [Y] then [ENTER]
Setup the correct permissons
[More to come on programming/setup, still writing it up!
]
TLDR: setting up a Raspberry Pi to monitor temperature, humidity, soil moisture and run a camera.
So currently running Raspbian GNU/Linux 10 (buster) on a Pi Model 3B with 1GB memory although it could probably run off Pi Zero WH ($16.58 USD). Any would do, as long as it has GPIO to connect the sensors and in my case, the camera connector.
Hooked upto this is a camera, a temp/humidity sensor and a capacitive moisture/temp sensor (most available from PiHut). I think it's not polite to link in forum so I've included model no's etc for your googling pleasure
Humidity/Temp:
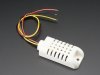
AM2302 (wired DHT22) temperature-humidity sensor ADA393 [AM2302] - $17.85 USD
Connectors:
Adafruit Premium Female/Female Jumper Wires - 20 x 12" (300mm) [ADA1949] - $5.10 USD
Soil sensor:
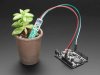
Adafruit STEMMA Soil Sensor - I2C Capacitive Moisture Sensor [ADA4026] - $9.56 USD
Connectors:
Adafruit JST PH 4-Pin to Female Socket Cable - I2C STEMMA Cable - 200mm [ADA3950] - $1.91 USD
Adafruit Premium Female/Male 'Extension' Jumper Wires - 20 x 12" [ADA1952] - $5.10 USD
Camera:
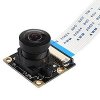
SainSmart Wide Angle Fish-Eye Camera Lenses for Raspberry Pi Arduino (approx $27 USD from amazon)
- Specs
- 5 MP Omnivision 5647 Camera Module
- Still Picture Resolution: 2592 x 1944
- Viewing angle: 160 degrees
- Video: Supports 1080 p @ 30 fps, 720 p @ 60 fps and 640 x480 p 60/90 Recording 15-pin MIPI Camera Serial Interface
- Mounting
- Adafruit Raspberry Pi Camera Board Case with 1/4" Tripod Mount [ADA3253] - $3.82 USD
- Handlebar For GoPro Hero Camera Seatpost Clamp Roll Bar Mount Adapter (approx $6 USD from ebay)
- Connectors
- Dorhea 6.56ft (2m) Raspberry Pi 3 B+ Camera Cable
C FPC Ribbon 15 pin Extension Cord Flat Wire Cable Connection for Raspberry Pi 2/3 B B+ Camera Module (approx $6 USD)
- Dorhea 6.56ft (2m) Raspberry Pi 3 B+ Camera Cable
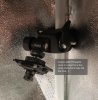
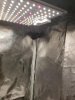
Wiring:
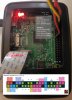
Hooking it all up:
I'll start with the two sensors.
Quick version:
Use python script to obtain readings every (x) seconds and send/save readings somewhere.
Run python script as a service so it runs on boot ..24/7
What you do with the readings can obviously vary a lot. Save them to a text file, a database etc on the Pi SD card or send them somewhere else over wifi/network.
Personally I prefer not relying on the SD card as they can be prone to failure and I wanted to be able to monitor them using apps I felt were a bit too demanding for running on the Pi (you could run them on the Pi though).
I instead opted to send the readings over http to InfluxDB which runs on a small linux NAS box at home. This means the Pi just has to get the readings and make an http request with the values which I can then view as my fancy looking dashboard.
There are quite a few good tutorials and variations on setting this up if you search for "am2302 raspberry pi influxdb"
The script I run for both sensors.
There are a few things to install which the script will need. To install them, run the following from the Pi's terminal. [Disclaimer: following is not complete at present]
Code:
cd /home/Pi
sudo apt-get update
sudo apt-get install build-essential python-dev python-openssl git
git clone https://github.com/adafruit/Adafruit_Python_DHT.git && cd Adafruit_Python_DHT
sudo python setup.py install
NB: when installing, you will be asked to confirm Y/N.
So first, create a new python script (called mine sensor.py) and saved it in a new folder (tent) under the default Pi user's home dir (/home/Pi/tent)
Code:
[/SIZE]
#!/usr/bin/python3
import time
import sys
import datetime
import Adafruit_DHT
import requests
import logging
from board import SCL, SDA
import busio
from adafruit_seesaw.seesaw import Seesaw
# Configure InfluxDB connection variables
interval = 12 # Sample period in seconds
host = "" # InfluxDb
port = 9999 # api ingestion port
org = ""
bucket = ""
url = f'http://{host}:{port}/api/v2/write?org={org}&bucket={bucket}&precision=s'
token = "" # user Pi
headers = { 'Authorization': f'Token {token}' }
# Enter the sensor details
sensor = Adafruit_DHT.DHT22
sensor_gpio = 4
i2c_bus = busio.I2C(SCL, SDA)
ss = Seesaw(i2c_bus, addr=0x36)
# think of measurement as a SQL table, it's not...but...
measurement = "rpi-dht22"
# location will be used as a grouping tag later
location = "tent"
prevTemp = -9999
prevHum = -9999
def get_change(current, previous):
if previous is None:
return 0
if current == previous:
return 0
try:
return (abs(current - previous) / previous) * 100.0
except ZeroDivisionError:
return None
# Run until you get a ctrl^c
try:
while True:
try:
# Read the sensor using the configured driver and gpio
humidity, temperature = Adafruit_DHT.read_retry(sensor, sensor_gpio)
moisture = ss.moisture_read()
soilTemp = ss.get_temp()
iso = int(time.time())
# odd reading is wild, if change is greater than 20% from last value, we skip
if (humidity is not None) and ((prevTemp == -9999 or prevHum == -9999) or (humidity < 100 and get_change(temperature, prevTemp) < 20 and get_change(humidity, prevHum) < 20)) :
# Print for debugging, uncomment the below line
# print("[%s] Temp: %s, Humidity: %s" % (iso, temperature, humidity))
prevTemp = temperature
prevHum = humidity
# send the data
dataT = f'{measurement},location={location} temperature={temperature},humidity={humidity},soilTemp={soilTemp},soilMoisture={moisture} {iso}'
response = requests.post(url, headers=headers, data=dataT, timeout=5.00)
except Exception:
logging.exception('Failed to process DHT reading')
pass
# Wait until it's time to query again...
time.sleep(interval)
except KeyboardInterrupt:
pass
[SIZE=4]
Once you have saved your script. The next step is to run it as a service.
Code:
cd /lib/systemd/system/
sudo nano tent.service
Then paste the following into the file.
Code:
[Unit]
Description=Tent monitoring
After=network-online.target
[Service]
ExecStart=/home/pi/tent/sensor.py
User=pi
[Install]
WantedBy=multi-user.target
You can save and exit the nano editor using [CTRL-X], [Y] then [ENTER]
Setup the correct permissons
Code:
sudo chmod 644 /lib/systemd/system/tent.service
sudo systemctl daemon-reload
sudo systemctl enable tent.service
sudo reboot
[More to come on programming/setup, still writing it up!
Last edited: